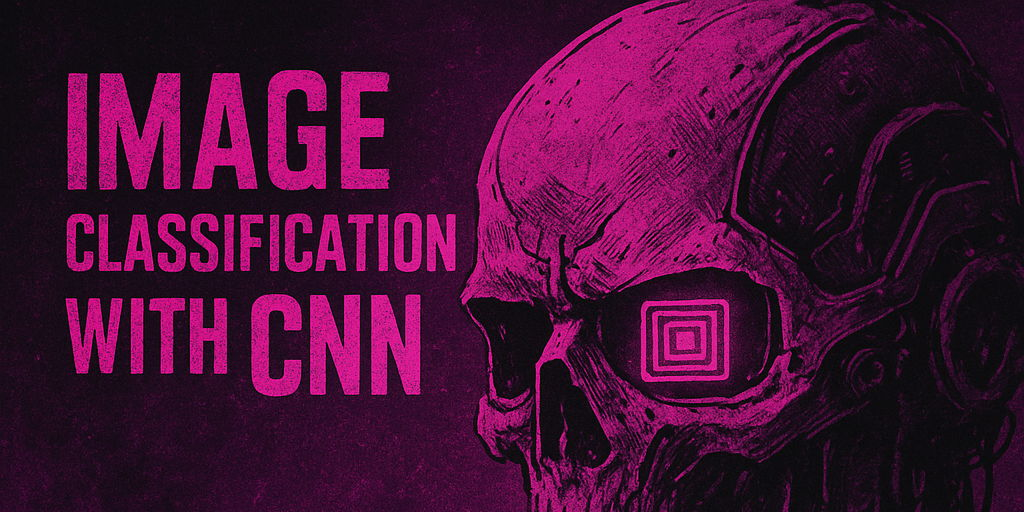
From MLP to CNN. Neural Networks for MNIST Digit Recognition
We build and compare four neural network architectures in PyTorch, visualize performance, explore complexity vs. accuracy, and show why CNNs excel at image classification.
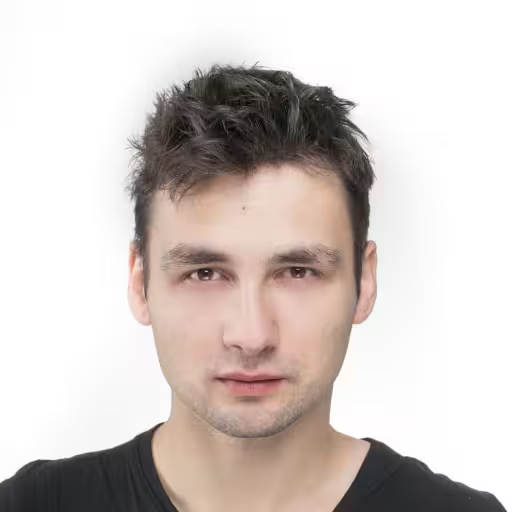
Daniel Gustaw
• 13 min read