Overload Signatures in Typescript
In TypeScript, we can specify a function that can be called in different ways by writing overload signatures. You can use this to define functions with returned type dependent from arguments values.
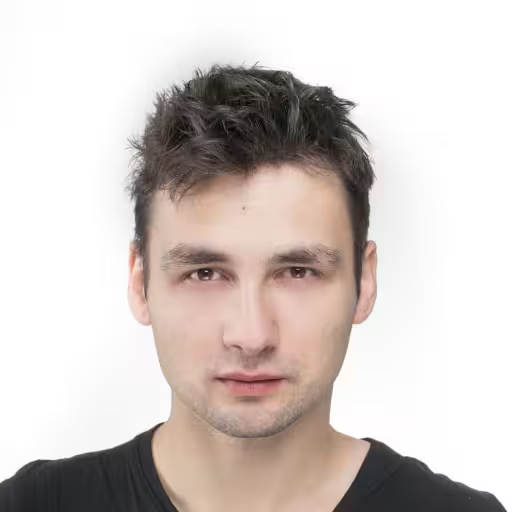
Daniel Gustaw
• 2 min read
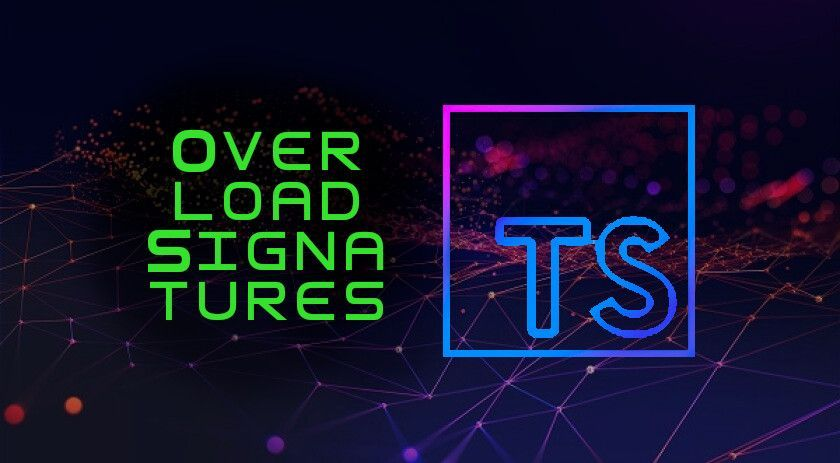
When do you have function that returns different types in dependence from parameter values overload signatures
can be exactly what do you need.
Let me introduce context.
We have super simple function that computes volume of hypercube:
interface HyperCube {
size: number
dimension: number
}
export function volume(cube: HyperCube): number {
return Math.pow(cube.size, cube.dimension);
}
But because our volumes are really huge, we need to display them in snake case notation. Eg.: 1_000_000_000
instead of 1000000000
.
We can add function to format
export function formatNumber(num: number): string {
return num.toString().replace(/(\d)(?=(\d{3})+(?!\d))/g, '$1_')
}
but we do not want to write this formatNumber
function always when we using conversion to string. Instead we would like to add second parameter to volume
function to decide if we returning string or number.
export function volume(cube: HyperCube, asString: boolean = false): string | number {
const volume = Math.pow(cube.size, cube.dimension);
return asString ? formatNumber(volume) : volume;
}
Unfortunately now using volume
function we do not know if we will get string
or number
. We do not want to use .toString
or parseInt
any time.
Fortunately, there is a concept called overload signatures. It allows selecting a returned type in dependence from parameters values.
In our case we want number
is asString
is false, in other case we need return string
. To apply overload signature we can use the following syntax
export function volume(cube: HyperCube, asString: false): number
export function volume(cube: HyperCube, asString: true): string
export function volume(cube: HyperCube, asString: boolean = false): string | number {
const volume = Math.pow(cube.size, cube.dimension);
return asString ? formatNumber(volume) : volume;
}
now our returned type is correct and depend from asString
value.
Sources:
Other articles
You can find interesting also.
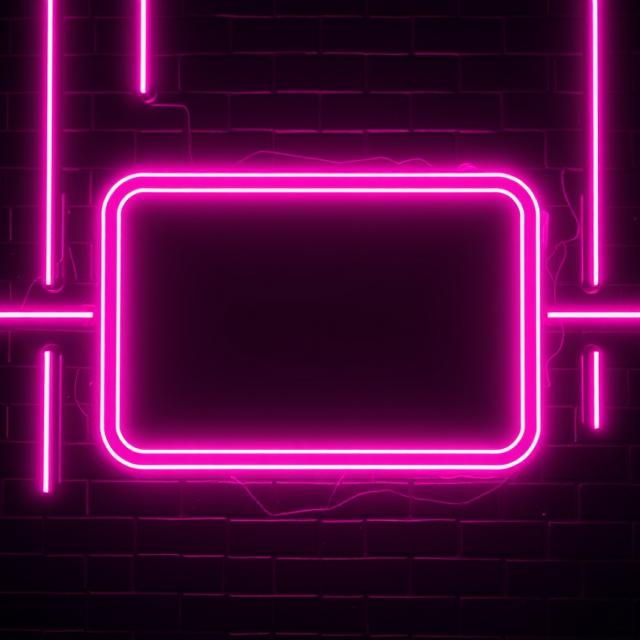
Web Push Notifications
Web push notification written in raw JavaScript without any libraries.
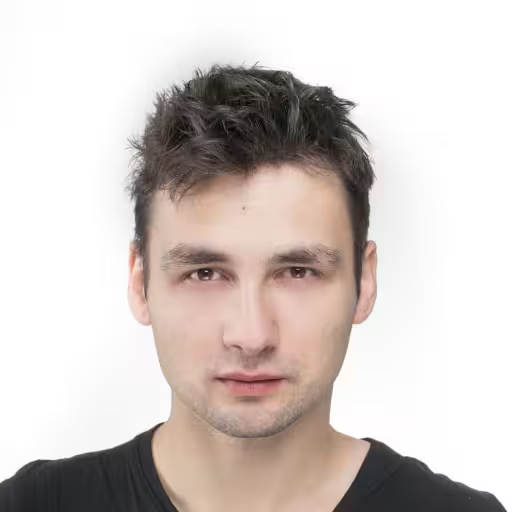
Daniel Gustaw
• 2 min read
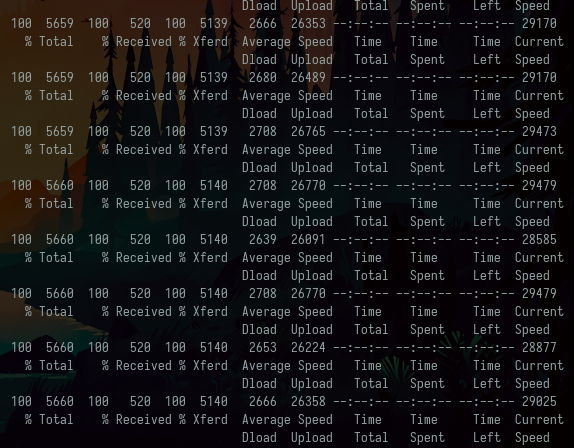
Scraping of the Pharmacy Register
Data administrators hate it. See how by entering two commands in the console he downloaded the register of all pharmacies in Poland.
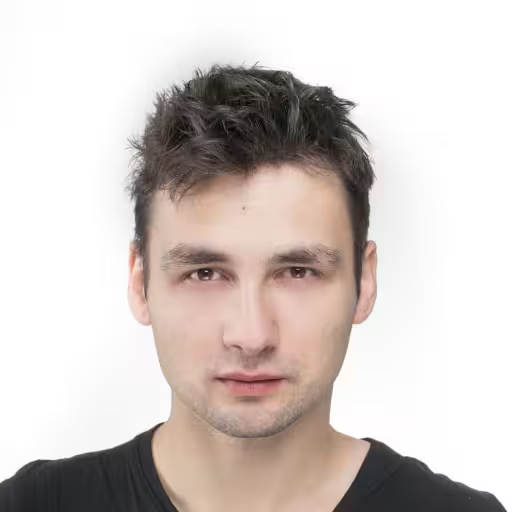
Daniel Gustaw
• 7 min read
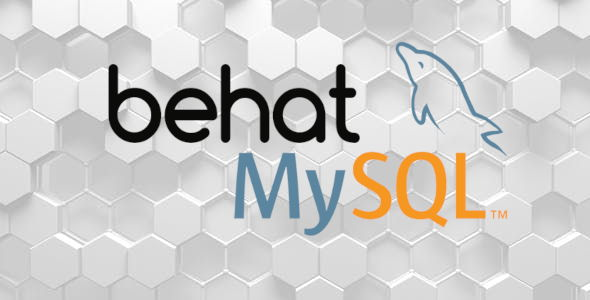
Tesseract-OCR and testing selects.
We will read the content of the database table from the photo and write a few tests for database queries in Behat.
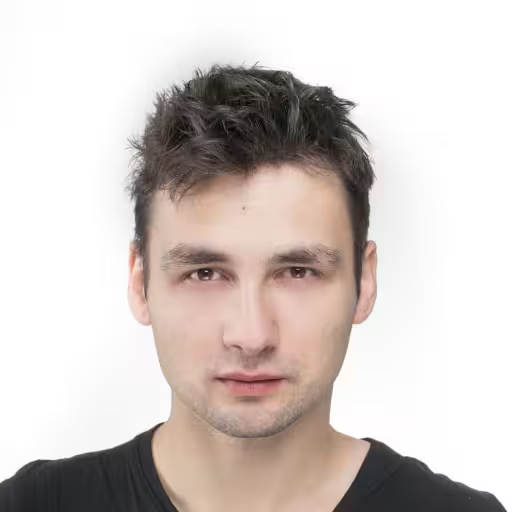
Daniel Gustaw
• 25 min read